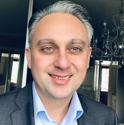
Python's Class Development Toolkit
I completed a tutorial by Raymond Hettinger “Python's Class Development Toolkit” on YouTube. The major takeaway from this excellent tutorial: • In Python it is normal to expose your attribute. When you expose an attribute expect users to do all kinds of interesting things with it. • Customers change the functionality of your code usually through subclassing. Customers can overwrite the parent class's method by writing the same named method in their subclass. If the parent method is called in subclass - it is called extending. If the parent method is not called in the subclass - it is called overwriting. • When there is a constructor war everyone should win provided the alternative constructors. The technique for doing this is @classmethod, parameter to it is 'cls', be sure you use this parameter because it will support subclassing. • If the class function doesn't need to know anything about the instance use @staticmethod on it. The purpose of the @staticmethod is to attach functions to classes. Use it to improve the findability of a function. • Micromanagement: e.g. government request: they tell you how to do your product. • The purpose of double underscore, what is called a class local reference, we are to make sure that self is actually refers to the parent class and not to self in a subclass. Most of the time self means the parent or your children. But occasionally it needed to be only the parent self. Thus, the double underscore is used! The intention of the double underscore is that self refers to you and not to a child self subclass. Double underscore will name mangle for parent self and rename this variable to '_parentClassName__variable' and if subclass will also make double underscore for the same variable it will not overwrite parent variable but simply Python will name mangle and rename it to '_childClassName__variable'. Double underscore is about freedom. It makes your subclass free to over-cry any other method without breaking any others. • @property's getter and setter convert dotted attribute access to method calls. This allows exposing our attribute and if we may need to fix this attribute later we use @property and the code remains with no changes. • Optimization tool when scaling up to a million of instances: '__slots__' is a flyweight design pattern that suppresses the instance dictionary and frees up space. It shrinks the size of the instance. Do __slots__ at the end of the project. __slots__ don't affect subclass attributes. • Agile and Lean program management. • Nice to be fully documented as you can run it through Spinx and make a pdf out of it for the users and managers. You can find this tutorial at: https://www.youtube.com/watchv=HTLu2DFOdTg&ab_channel=NextDayVideo