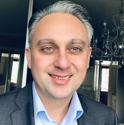
Logging in Python
I completed two tutorials on Logging in Python. o The first tutorial is “Python Tutorial: Logging Basics - Logging to Files, Setting Levels, and Formatting and Python Tutorial: Logging Advanced - Loggers, Handlers, and Formatters” instructed by Corey Schafer, he is a full-time content creator publishing regular Python tutorials on YouTube. The length of the tutorial is 0.30 total video hours. o The second tutorial is “Logging in Python” offered by Real Python and instructed by Abhinav Ajitaria. You can find these tutorials at · https://www.youtube.com/watch?v=-ARI4Czawo&ab_channel=CoreySchafer · https://www.youtube.com/watch?v=jxmzY9soFXg&ab_channel=CoreySchafer · https://realpython.com/python-logging/