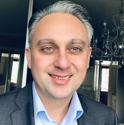
Create an Executable File from Python File using Pyintaller library
I learned how to create from `.py` files `.exe` files to make applications, using `pyinstaller` library and `auto-py-to-exe` library. `auto-py-to-exe` library also uses `pyinstaller` in the background but has a helpful interface. Additionally, I learned: o How to analyze error log files like warn-main.txt. o Where to find hooks and libraries that are recognized by default by pyinstaller. o What to do if something goes wrong during the creation of `.exe` file like: • in case of pyinstaller cannot find some libraries use `--hidden-import=module_name` flag • in case of pyintaller cannot find some path to outside image files use a. `--additional-hooks-dir=file_name.jpg` flag b. create relative path function that will find the path to the file • in case of pyinstaller cannot recognize the relative path to import the module from another Python file use `--paths "path_to_the_file" flag o Finally, I learned how to make my executable file ready for sharing with others via email. I used NSIS tool that creates a single file that bundles up all the dependencies under one file.