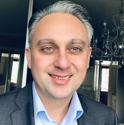
Statistics in Python - Generating Random Numbers in Python, NumPy, sklearn, and seaborn
In a Deep Dive course Part I by Fred Baptiste I learned about how to generate random numbers and use seeds, choice, and sample. However, I wanted to get a deeper understanding of the subject. Therefore, I took additional three tutorials on this subject. o The first intermediate-level tutorial I completed is the “Generating Random Data in Python (Guide)” offered by Real Python. The instructor of the tutorial is Brad Solomon. In this tutorial I learned about: • How Random is Random? • Cryptographically Secure Pseudorandom Number Generator (CSPRNG) – os.urandom(), secret, uuid • Pseudorandom Number Generator (PRNG) • PRNG vs TRNG • PRNG vs CSPRNG • Use numpy.random and numpy.set_printoptions() You can find this tutorial at https://realpython.com/python-random/ o The second intermediate-level tutorial I completed is the “Statistics in Python — Generating Random Numbers in Python, NumPy, and sklearn” offered by Towards Data Science dot com. The instructor of the tutorial is Wei-Meng Lee. In this tutorial I learned about: • How to use matplotlib to visualize distributions • Generate random numbers in Python • Uniform distribution • Normal or Gaussian distribution • Linearly distribution using sklearn 2D plot • Linearly Distribution using sklearn 2D plot Interpolation • Linearly Distribution using sklearn 3D plot • Generating random numbers that cluster around centroids 2D • Generating random numbers that cluster around centroids 3D • Generating Correlated Data • Seeding your Random Number Generator You can find this tutorial at https://towardsdatascience.com/statistics-in-python-generating-random-numbers-in-python-numpy-and-sklearn-60e16b2210ae o The third intermediate-level tutorial I completed is the “Normal Distribution Vs Uniform Distribution” taught by Ankit at “thatascience”. It provided the code for generating and plotting Normal vs Uniform distribution using Numpy, matplotlib, seaborn and random. You can find this tutorial at https://thatascience.com/learn-numpy/normal-vs-uniform/