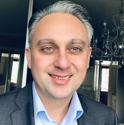
Certificate of Completion - Practical Python unit testing with Pytest + mocking |Pycharm
I earned a Certificate of Completion that verifies that I successfully completed the beginner-level “Practical Python unit testing with Pytest + mocking |Pycharm” course on 11/28/2021 as taught by instructor Faisal Memon at Udemy Academy. Faisal Memon is a Google Launchpad Accelerator. The certificate indicates the entire course was completed as validated by the student. The course duration represents the total video hours of the course at the time of most recent completion. Length 3.5 total hours. What I learned in the course: • Assertion • Fixtures and yield with fixtures • Test exceptions • Markers – xfail, skip, parameterized • Learn about mocking and how to create mocks to cut down dependencies – parameterized, verifying mocks, mocking JSON values • Learn how can you mock APIs and verify it You can find this course at https://www.udemy.com/course/practical-unit-testing-for-python-with-pytest-and-mocking/